Data Structures and Algorithms (DSA) is the study of methods for organizing and storing data in a computer and the design of procedures or formulas (algorithm) for solving problems, often manipulating the data stored in the data structures. If you’ve ever wondered why some programs run faster or why certain apps perform better, the answer lies in DSA. DSA is one of the most important and must have skill for every computer science student. By choosing right data structure and algorithm, you can optimize your program to run faster, especially when handling large datasets.
By mastering DSA, you will not only become a better programmer, but also increase your chance to perform well in job interviews in top tech companies. A strong understanding of DSA often leads you to landing roles at tech giants like Google, Microsoft, Amazon, Meta (formerly Facebook), etc.
This DSA tutorial will guide you through the essentials, helping you quickly and effectively improve your skills in problem solving and algorithm design.
What Are Data Structures?
We first try to understand the concept with a real-life example. Let’s imagine you’re in your study room. There, all books are scattered in the desk. Feels like a jungle, right? Hahaha. Now, if someone asks you to give a certain book, can you find it quickly? Not at all.
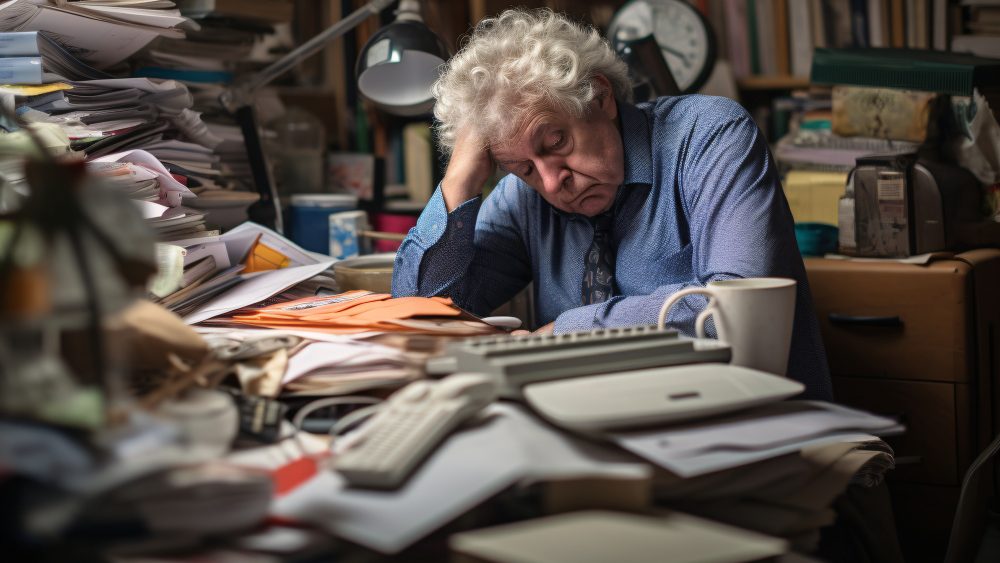
Let’s organized the books in stacks.
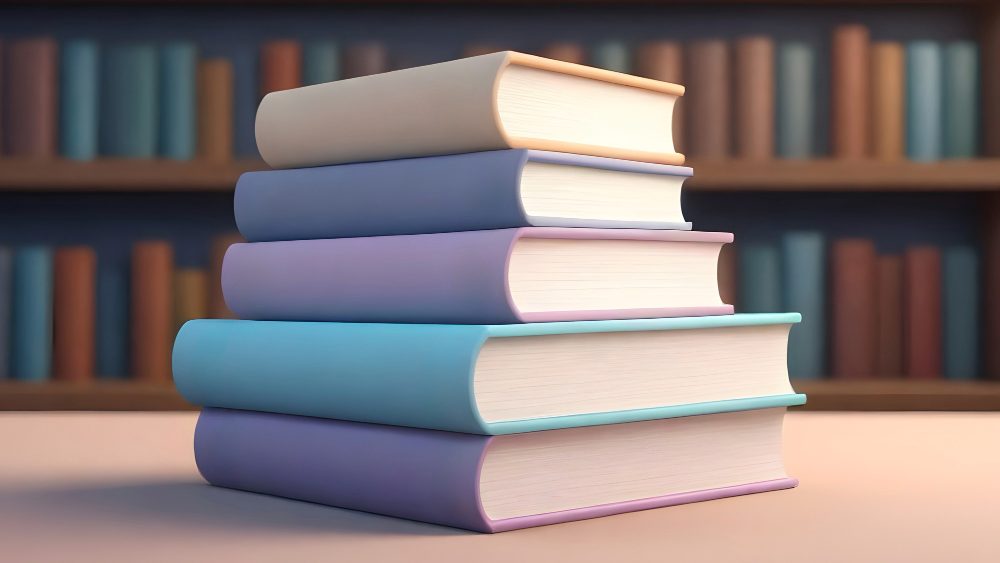
The desk is now clean and more accessible, right? To find a certain book, you can just look for its name easily.
Now, imagine a library with thousands of books, organized in shelfs. Each shelf is labeled with a letter. Now, to find a book, “Data Structures and Algorithms (DSA) – by HowToDSA”, what shelf will you look? Of course the one that has the letter “D”?
Think, how complex it would be if the books weren’t orgranized?
Here, the structure is very similar to a data structure. In computer, we organize and store data in these data structures and retrieve the data from these data structures when needed.
There are many data structures having their own use cases. Each has also its own pros and cons. Some data structures are simple, like Arrays (a list of items), and some are a bit more advanced, like Trees (which organize data in a hierarchy). Though not all data fits in one single data structure. Rather we, the programmers need to choose the right data structure accordingly.
Some common Data Structures include:
- Arrays: A simple list of items.
- Linked Lists: A chain of items where each item points to the next one.
- Stacks: Like a stack of books where you can only take the top one off.
- Queues: Like a line of people where the first person in line is served first.
These are the building blocks we’ll work with when solving programming problems. In fact, data structures are the essential ingredients in creating fast and powerful algorithms. Choosing the right one helps in organizing and managing data, reduce complexity, and increase efficiency.
What Are Algorithms?
An Algorithm is just a fancy name of a set of steps to solve a problem. It tells us how to do a certain work and achieve the desired result. To understand what an algorithm is, let’s think of how we make coffee.
- Step 1: Boil water in a kettle.
- Step 2: Add coffee grounds to a filter in the coffee maker.
- Step 3: Pour the boiling water over the coffee grounds.
- Step 4: Brew for a few minutes and pour into a cup and enjoy!
This step-by-step process for making coffee serves as a perfect example of an algorithm. Characterized by its clear and logical sequence of instructions, an algorithm is designed to achieve specific goals.
When discussing algorithms in computer science, we typically write step-by-step instructions in a programming language such as C, C++, Java or Python. Moreover, rather than the ingredients, algorithms use data structures.
In programming, algorithms do things like:
- Search for a specific item in a list.
- Sort items, like arranging numbers from smallest to largest.
- Find the shortest path between two points, which is how map apps help you navigate.
How DSA Works Together
Imagine you have a huge list of names and you want to find one specific name quickly from there. If you use an efficient data structure, like an array, and a clever algorithm, like binary search, you can find that name in no time! That’s the power of using DSA – the right data structure makes organizing information easy, and the right algorithm helps you solve the problem efficiently.
Real World Examples of DSA
To help you understand the usage of DSA clearly, here are some everyday applications:
- Search Engines like Google use algorithms to quickly search through billions of web pages and find the most relevant result for your query.
- Social Media platforms like Facebook organize your friend list and posts using different data structures so that you can find and interact with them easily.
- Navigation Apps like Google Maps use algorithms to find the shortest route from your location to your destination.
How Will This Tutorial Help You?
This DSA tutorial series will help you learn the basics, master intermediate topics and then prepare for coding interviews. We will start with simple concepts like arrays, linked lists, stacks, and queues. Once you understand the basics, we will dive into more advanced topics like searching, sorting, and dynamic programming. Finally, if you aim to land a job at a top tech company, these tutorials will give you the tools you need to undergo the technical interviews.
What’s next?
We will start with Big-O Notation, which is a simple way to measure how fast (or slow) an algorithm works. Understanding this concept will help you to choose the right algorithm for different solutions. Stay tuned, and let’s continue this exciting journey into the world of DSA!